Declaration, initialization and usage of pointers
C program to declare, initialize and depict usage of the pointers.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int i, *j, **k;
i = 10;
j = &i;
k = &j;
printf("\tValues in variables\n");
printf("Value in i %d\n",i);
printf("Value in j (which is the address of i) %d\n",j);
printf("Value in k (which is the address of j) %d\n",k);
//Lets see what is the real address of i,j,k
printf("\n\tAddresses\n");
printf("Address of i (which is the value in j) %u\n",&i);
printf("Address of j (which is the value in k) %u\n",&j);
printf("Address of k %u\n",&k);
//printing using pointer
printf("\n\tValues using pointers\n");
printf("Value in i %d\n",*(&i));
printf("Value in i %d\n",*j);
printf("Value in i %d\n",**k);
printf("Value in j %d\n",*(&j));
printf("Value in j %d\n",*k);
printf("Value in k %d\n",*(&k));
getch();
}
Output
Values in variables
Value in i 10
Value in j (which is the address of i) 6422300
Value in k (which is the address of j) 6422296
Addresses
Address of i (which is the value in j) 6422300
Address of j (which is the value in k) 6422296
Address of k 6422292
Values using pointers
Value in i 10
Value in i 10
Value in i 10
Value in j 6422300
Value in j 6422300
Value in k 6422296
Explanation
Pointer Declaration
A pointer variable holds memory address of some other variable instead of holding the actual value itself.
Syntax to declare pointer variable:
Data type of a pointer must be same as the data type of the variable to which the pointer variable is pointing.
For example,
int *num; //pointer to integer variable
float *price; //pointer to float variable
char *ch; //pointer to character variable
Pointer Initialization
Pointer Initialization is the process of assigning address of a variable to a pointer variable. Pointer variable can only contain address of a variable of the same data type. In C language address operator & is used to determine the address of a variable. The & (immediately preceding a variable name) returns the address of the variable associated with it.
Let us understand this with the help of an example.
Here, 'i' is a normal variable and 'j' is a pointer variable.
variable j is storing the address of variable i (j = &i;)
Using the pointer (dereferencing pointer)
Once a pointer has been assigned the address of a variable, to access the value of the variable, pointer is dereferenced, using the indirection operator or dereferencing operator *.
Let us understand this with the help of an example.
To access the value of a certain address stored by a pointer variable, * is used. Here, the * can be read as 'value at'.
Pointer to a pointer
Pointers are used to store the address of other variables of similar datatype. But if you want to store the address of a pointer variable, then you again need a pointer to store it. Thus, when one pointer variable stores the address of another pointer variable, it is known as Pointer to Pointer variable or Double Pointer.
Syntax:
example,
Here, we have used two indirection operator(*) which stores and points to the address of a pointer variable i.e, int *.
If we want to store the address of this (double pointer) variable p1, then the syntax would become:
Now, In the above program, a normal variable 'i', a pointer variable 'j' and a double pointer variable 'j' are declared.
Here, 'i' is a normal integer variable, which contains the value 10
'j' is a pointer variable that stores the address of a normal integer variable i.e. 'i'.
'k' is a double pointer variable that stores the address of a pointer integer variable i.e. 'j'.
This statement simply prints the value of i i.e. 10.
This statement prints the value in pointer variable j, which is 6422300 (address of i) as shown in the above figure.
This statement prints the value in pointer variable k, which is 6422296 (address of j) clearly depicted in the above figure.
This statement prints the address of variable i, which is 6422230.
This statement prints the address of pointer variable j, which is 6422296.
This statement prints the address of pointer variable k, which is 6422292.
A pointer variable holds memory address of some other variable instead of holding the actual value itself.
Syntax to declare pointer variable:
<data_type> *<variable_name>;
Data type of a pointer must be same as the data type of the variable to which the pointer variable is pointing.
For example,
int *num; //pointer to integer variable
float *price; //pointer to float variable
char *ch; //pointer to character variable
Pointer Initialization
Pointer Initialization is the process of assigning address of a variable to a pointer variable. Pointer variable can only contain address of a variable of the same data type. In C language address operator & is used to determine the address of a variable. The & (immediately preceding a variable name) returns the address of the variable associated with it.
Let us understand this with the help of an example.
int i;
int *j;
//pointer declaration
j = &i;
//pointer initialization
Here, 'i' is a normal variable and 'j' is a pointer variable.
variable j is storing the address of variable i (j = &i;)
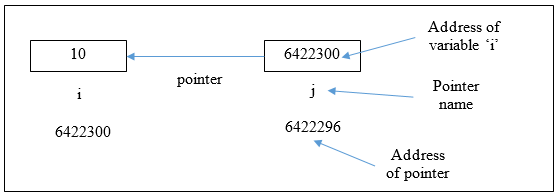
Using the pointer (dereferencing pointer)
Once a pointer has been assigned the address of a variable, to access the value of the variable, pointer is dereferenced, using the indirection operator or dereferencing operator *.
Let us understand this with the help of an example.
int i;
int *j;
//pointer declaration
j = &i;
//pointer initialization
i = 10;
printf("%d", *j);
//Dereferencing operator, will print the value of i i.e. 10
To access the value of a certain address stored by a pointer variable, * is used. Here, the * can be read as 'value at'.
Pointer to a pointer
Pointers are used to store the address of other variables of similar datatype. But if you want to store the address of a pointer variable, then you again need a pointer to store it. Thus, when one pointer variable stores the address of another pointer variable, it is known as Pointer to Pointer variable or Double Pointer.
Syntax:
<data-type> **<pointer-name>
example,
int **p1;
Here, we have used two indirection operator(*) which stores and points to the address of a pointer variable i.e, int *.
If we want to store the address of this (double pointer) variable p1, then the syntax would become:
int ***p2;
Now, In the above program, a normal variable 'i', a pointer variable 'j' and a double pointer variable 'j' are declared.
int i, *j, **k;
i = 10;
j = &i;
k = &j;
Here, 'i' is a normal integer variable, which contains the value 10
'j' is a pointer variable that stores the address of a normal integer variable i.e. 'i'.
'k' is a double pointer variable that stores the address of a pointer integer variable i.e. 'j'.
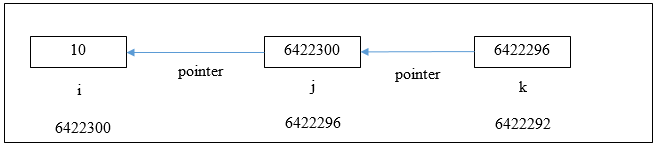
printf("Value in i %d\n",i);
This statement simply prints the value of i i.e. 10.
printf("Value in j (which is the address of i) %d\n",j);
This statement prints the value in pointer variable j, which is 6422300 (address of i) as shown in the above figure.
printf("Value in k (which is the address of j) %d\n",k);
This statement prints the value in pointer variable k, which is 6422296 (address of j) clearly depicted in the above figure.
printf("Address of i (which is the value in j) %u\n",&i);
This statement prints the address of variable i, which is 6422230.
printf("Address of j (which is the value in k) %u\n",&j);
This statement prints the address of pointer variable j, which is 6422296.
printf("Address of k %u\n",&k);
This statement prints the address of pointer variable k, which is 6422292.
printf("Value in i %d\n",*(&i));
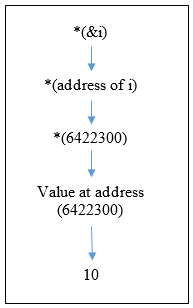
printf("Value in i %d\n",*j);
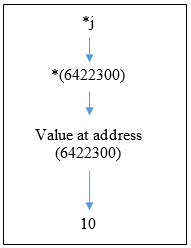
printf("Value in i %d\n",**k);
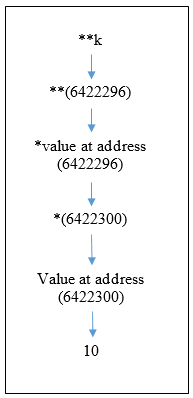
printf("Value in j %d\n",*(&j));
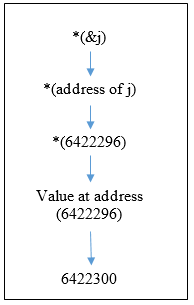
printf("Value in j %d\n",*k);
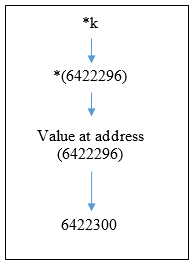
printf("Value in k %d\n",*(&k));
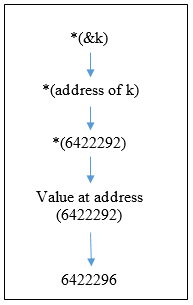