LCM of two numbers
C Program to Find LCM of two Numbers.
Least Common Multiple (LCM) of two or more integers is the smallest positive integer that is perfectly divisible by all the numbers without the remainder. In other words, we can say that, LCM is the smallest positive value which is multiple of all the numbers.
For example, LCM of 6 and 8
Multiples of 6 --> 6, 12, 18,
Multiples of 8 --> 8, 16,
The smallest multiple of both is 24, hence, the LCM of 6 and 8 is 24.
Mathematically, LCM can be calculated as follows:
We can also calculate LCM using GCD (shown in method 2)
LCM = (num1 * num2) / GCD
For example, LCM of 6 and 8
Multiples of 6 --> 6, 12, 18,
24
, 30, 36, 42, 48 ...
Multiples of 8 --> 8, 16,
24
, 32, 40, 48, 56, 64 ...
The smallest multiple of both is 24, hence, the LCM of 6 and 8 is 24.
Mathematically, LCM can be calculated as follows:
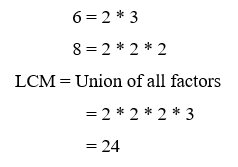
We can also calculate LCM using GCD (shown in method 2)
LCM = (num1 * num2) / GCD
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int num1, num2, lcm;
printf("Enter two positive integers: ");
scanf("%d%d",&num1,&num2);
// maximum number between num1 and num2 is stored in lcm
lcm = (num1>num2) ? num1 : num2;
// Always true
while(1)
{
if( lcm%num1==0 && lcm%num2==0 )
{
printf("The LCM of %d and %d is %d.", num1,num2,lcm);
break;
}
lcm++;
}
getch();
}
Output
Enter two positive integers: 12
80
The LCM of 12 and 80 is 240.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int num1, num2, lcm, i, gcd;
printf("Enter two positive integers: ");
scanf("%d%d",&num1,&num2);
for(i=1; i <= num1 && i <= num2; i++)
{
// Checks if i is factor of both integers
if(num1%i==0 && num2%i==0)
gcd = i;
}
lcm = (num1 * num2)/gcd;
printf("The LCM of %d and %d is %d.", num1,num2,lcm);
getch();
}
Output
Enter two positive integers: 12
82
The LCM of 12 and 82 is 492.