Roots of a quadratic equation
C program to find out the roots of a quadratic equation a*x*x + b*x + c = 0, where a, b, and c are entered by the user.
The standard form of a quadratic equation is:
The term
If the discriminant is greater than 0, the roots are real and different.
If the discriminant is equal to 0, the roots are real and equal.
If the discriminant is less than 0, the roots are complex (imaginary) and different.
ax2 + bx + c = 0, where
a, b and c are real numbers and
a != 0
The term
b2-4ac
is known as the discriminant of a quadratic equation. It tells the nature of the roots.
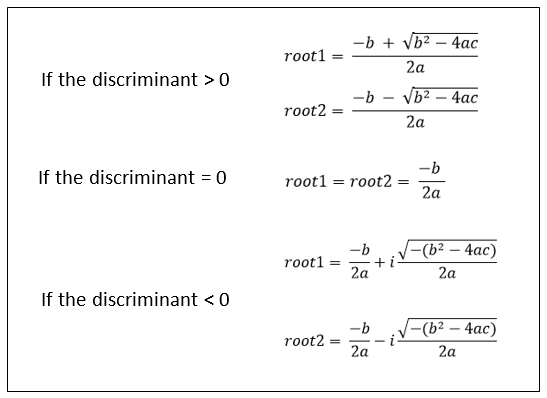
Program
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
#include<math.h>
void main()
{
float a, b, c;
float disc, root1, root2;
float temp1, temp2;
printf("\nEnter the values of a,b,c\t:");
scanf("%f%f%f",&a,&b,&c);
if(a==0)
{
printf("\nThe equation is linear and not quadratic");
getch();
exit(0);
}
disc = (b * b) - (4 * a * c);
if(disc < 0)
{
temp1= ((-1) * b) / (2 * a);
temp2= (sqrt((-1) * disc)) / (2 * a);
printf("\nEquation has imaginary roots and are\n1. %.2f + i%.2f\n2. %.2f - i%.2f",temp1,temp2,temp1,temp2);
}
else if(disc == 0)
{
root1 = ((-1) * b) / (2 * a);
root2 = root1;
printf("\nThe roots are equal and are\n1. %.2f\n2. %.2f",root1,root2);
}
else if(disc > 0)
{
root1 = (((-1) * b) + sqrt(disc)) / (2 * a);
root2 = (((-1) * b) - sqrt(disc)) / (2 * a);
printf("\nThe roots are real and are\n1. %.2f\n2. %.2f",root1,root2);
}
getch();
}
Output
********** Run1 **********
Enter the values of a,b,c :1
2
1
The roots are equal and are
1. -1.00
2. -1.00
********** Run2 **********
Enter the values of a,b,c :2
6
2
The roots are real and are
1. -0.38
2. -2.62
********** Run3 **********
Enter the values of a,b,c :1
1
1
Equation has imaginary roots and are
1. -0.50 + i0.87
2. -0.50 - i0.87
********** Run4 **********
Enter the values of a,b,c :0
3
4
The equation is linear and not quadratic
Explanation
Input coefficients of quadratic equation from user. Store it in some variable say a, b and c.
First of all, we will check whether the entered equation is quadratic or not. This is checked using the value of 'a' coefficient. If a = 0, then, it is not a quadratic equation but a linear equation. So, we have to exit the program there only. This is done using the exit(0) statement. This predefined function is defined in stdlib.h header file.
But if value of 'a' is non zero, then it is a quadratic equation and we have to calculate the roots of that equation.
Next step is to calculate discriminant of the quadratic equation
Now roots are calculated based on the nature of discriminant.
if
else if
else if
First of all, we will check whether the entered equation is quadratic or not. This is checked using the value of 'a' coefficient. If a = 0, then, it is not a quadratic equation but a linear equation. So, we have to exit the program there only. This is done using the exit(0) statement. This predefined function is defined in stdlib.h header file.
But if value of 'a' is non zero, then it is a quadratic equation and we have to calculate the roots of that equation.
Next step is to calculate discriminant of the quadratic equation
disc = (b * b) - (4 * a * c);
Now roots are calculated based on the nature of discriminant.
if
disc > 0
then,
root1 = (-b + sqrt(disc)) / (2*a)
root2 = (-b - sqrt(disc)) / (2*a)
else if
disc == 0
then,
root1 = root2 = -b / (2*a)
else if
disc < 0
then, there are two distinct complex roots,
root1 = -b / (2*a) + i (sqrt(-disc) / (2*a))
root2 = -b / (2*a) - i (sqrt(-disc) / (2*a))