Greater of two numbers using ternary (conditional) operator
C program to find greater of two numbers using conditional operator.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int num1, num2;
printf("Enter two numbers\t:");
scanf("%d%d",&num1,&num2);
(num1 > num2) ? printf("\nFirst number is greater") : printf("\nSecond number is greater");
getch();
}
Output
********** Run1 **********
Enter two numbers :4
7
Second number is greater
********** Run1 **********
Enter two numbers :8
3
First number is greater
Explanation
General Form of Ternary Operator (conditional operator):
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is executed/returned, and if expression_1 results in false then, expression_3 gets executed/returned.
In above program, we have to check that which of the two numbers entered by the user is greater.
Using conditional operator, this is done as follows:
If num1>num2 evaluates to true, then printf("\nFirst number is greater") statement is executed.
If num1>num2 evaluates to false, then printf("\nSecond number is greater") statement is executed.
(expression_1) ? (expression_2) : (expression_3);
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is executed/returned, and if expression_1 results in false then, expression_3 gets executed/returned.
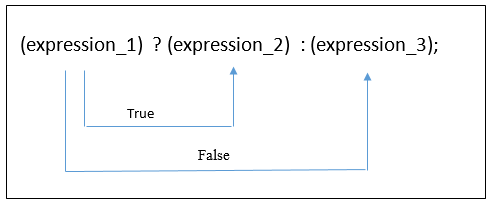
In above program, we have to check that which of the two numbers entered by the user is greater.
Using conditional operator, this is done as follows:
(num1 > num2) ? printf("\nFirst number is greater") : printf("\nSecond number is greater");
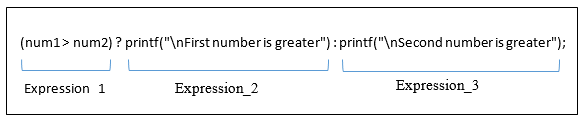
If num1>num2 evaluates to true, then printf("\nFirst number is greater") statement is executed.
If num1>num2 evaluates to false, then printf("\nSecond number is greater") statement is executed.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int num1, num2, max;
printf("Enter two numbers\t:");
scanf("%d%d",&num1,&num2);
max = (num1 > num2) ? num1 : num2;
printf("Maximum number between %d and %d is %d", num1, num2, max);
getch();
}
Output
Enter two numbers :4
7
Maximum number between 4 and 7 is 7
Explanation
Another Form of Ternary Operator (conditional operator):
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is returned and stored in the variable, and if expression_1 results in false then, expression_3 is returned and stored in the variable.
In above program, we have to check that which of the two numbers entered by the user is greater. Using conditional operator, this is done as follows:
If num1>num2 evaluates to true, then num1 is returned and max = num1.
If num1>num2 evaluates to false, then num2 is returned and max = num2.
Variable = (expression_1) ? (expression_2) : (expression_3);
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is returned and stored in the variable, and if expression_1 results in false then, expression_3 is returned and stored in the variable.

In above program, we have to check that which of the two numbers entered by the user is greater. Using conditional operator, this is done as follows:
max = (num1 > num2) ? num1 : num2;

If num1>num2 evaluates to true, then num1 is returned and max = num1.
If num1>num2 evaluates to false, then num2 is returned and max = num2.