Greatest of three numbers using conditional (ternary) operator
C program to find the greatest of three numbers using Conditional (ternary) Operator.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
int num1, num2, num3;
printf("\nEnter the first number\t:");
scanf("%d",&num1);
printf("\nEnter the second number\t:");
scanf("%d",&num2);
printf("\nEnter the third number\t:");
scanf("%d",&num3);
(num1>num2) ? ((num1>num3) ? printf("\n%d is greatest",num1) : printf("\n%d is greatest",num3)) : ((num2>num3) ? printf("\n%d is greatest",num2) : printf("\n%d is greatest",num3));
getch();
}
Output
********** Run1 **********
Enter the first number :12
Enter the second number :23
Enter the third number :21
23 is greatest
********** Run2 **********
Enter the first number :23
Enter the second number :34
Enter the third number :45
45 is greatest
********** Run3 **********
Enter the first number :34
Enter the second number :32
Enter the third number :21
34 is greatest
Explanation
Three numbers are taken as input from the user in the variables named 'num1', 'num2' and 'num3' respectively.
First of all, condition
In Expression_2, condition
Similarly, in Expression_3, condition
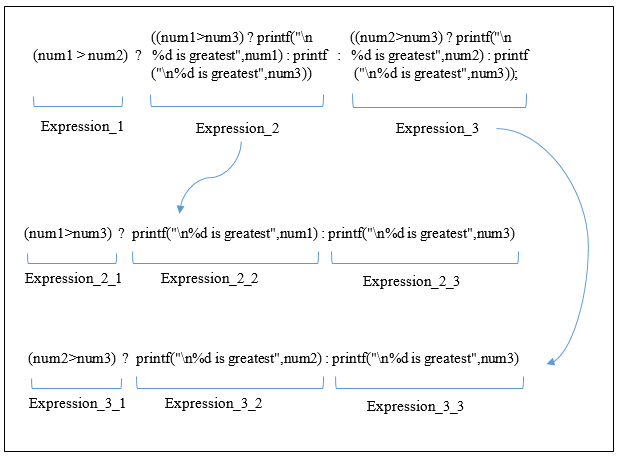
First of all, condition
(num1 > num2)
is checked. If the condition results in true, then Expression_2 is executed and if the condition results in false, then Expression_3 is executed.
In Expression_2, condition
(num1 > num3)
is checked. If the condition results in true, then Expression_2_2 is executed and if the condition results in false, then Expression_2_3 is executed.
Similarly, in Expression_3, condition
(num2 > num3)
is checked. If the condition results in true, then Expression_3_2 is executed and if the condition results in false, then Expression_3_3 is executed.