Vowel or not using conditional (ternary) operator
C program to determine whether the character entered is vowel or not using conditional (ternary) operator.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
char ch;
printf("Enter a character\t:");
scanf("%c",&ch);
(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U') ? printf("\nEntered character is a vowel") : printf("\nEntered character is not a vowel");
getch();
}
Output
********** Run1 **********
Enter a character :g
Entered character is not a vowel
********** Run2 **********
Enter a character :E
Entered character is a vowel
Explanation
General Form of Ternary Operator (conditional operator):
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is executed/returned, and if expression_1 results in false then, expression_3 gets executed/returned.
To check whether a character is a vowel, we have to check for both uppercase and lowercase vowels i.e. a,e,i,o,u,A,E,I,O and U
Condition for checking is
Note that Character in C is represented inside single quote. Do not forget to add single quote whenever checking for character constant.
Using conditional operator, this is done as follows:
If entered character is among these alphabets (a, e, i, o, u, A, E, I, O, U) then condition in expression_1 evaluates to true, and, statement in expression_2
If entered character is not one of these alphabets the condition evaluates to false, and, statement in expression_3
(expression_1) ? (expression_2) : (expression_3);
expression_1 is a comparison/conditional argument. If expression_1 results in true then, expression_2 is executed/returned, and if expression_1 results in false then, expression_3 gets executed/returned.
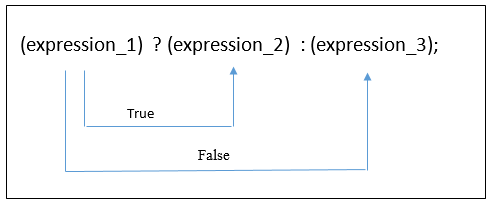
To check whether a character is a vowel, we have to check for both uppercase and lowercase vowels i.e. a,e,i,o,u,A,E,I,O and U
Condition for checking is
if(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U')
Note that Character in C is represented inside single quote. Do not forget to add single quote whenever checking for character constant.
Using conditional operator, this is done as follows:
(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U') ? printf("\nEntered character is a vowel") : printf("\nEntered character is not a vowel");
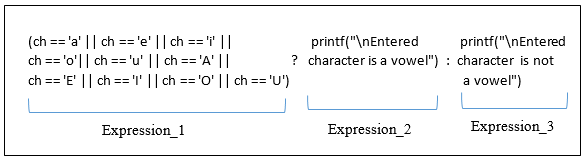
If entered character is among these alphabets (a, e, i, o, u, A, E, I, O, U) then condition in expression_1 evaluates to true, and, statement in expression_2
printf("\nEntered character is a vowel")
is executed.
If entered character is not one of these alphabets the condition evaluates to false, and, statement in expression_3
printf("\nEntered character is not a vowel")
is executed.