Sum of digits of five digit number
If a five-digit number is input through the keyboard, write a C program to calculate the sum of its digits.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
long int num, sum = 0;
printf("Enter a five digit number\t:");
scanf("%ld", &num);
sum = sum + num%10;
num = num/10;
sum = sum + num%10;
num = num/10;
sum = sum + num%10;
num = num/10;
sum = sum + num%10;
num = num/10;
sum = sum + num%10;
printf("\nSum of digits = %d",sum);
getch();
}
Output
Enter a five digit number :56432
Sum of digits = 20
Explanation
A five digit number is taken as an input from the user in the variable named 'num'. %ld is the format specifier used for long int data type.
A variable named 'sum' is used to carry the sum of the digits of the number. sum is initialized to 0.
For example, if num = 56432
then, num % 10 = 2
This digit is added to the sum variable using statement:
sum = sum + num % 10;
sum = 0 + 2
sum = 2
Now, we have to add the remaining digits to this variable.
num = 56432
then, num / 10 = 5643
Now, the above procedure is repeated 5 times, until all the digits are added to variable 'sum'.
sum = 0
num = 56432
sum = 20
A variable named 'sum' is used to carry the sum of the digits of the number. sum is initialized to 0.
num%10
operation extracts the digit of unit place.
For example, if num = 56432
then, num % 10 = 2
This digit is added to the sum variable using statement:
sum = sum + num % 10;
sum = 0 + 2
sum = 2
Now, we have to add the remaining digits to this variable.
num/10
operation extracts the number except the digit in unit place.
num = 56432
then, num / 10 = 5643
Now, the above procedure is repeated 5 times, until all the digits are added to variable 'sum'.
Dry run:
sum = 0
num = 56432
sum = sum + num%10;
sum = 0 + 56432%10 = 0 + 2 = 2
num = num/10;
num = 56432/10 = 5643
sum = sum + num%10;
sum = 2 + 5643%10 = 2 + 3 = 5
num = num/10;
num = 5643/10 = 564
sum = sum + num%10;
sum = 5 + 564%10 = 5 + 4 = 9
num = num/10;
num = 564/10 = 56
sum = sum + num%10;
sum = 9 + 56%10 = 9 + 6 = 15
num = num/10;
num = 56/10 = 5
sum = sum + num%10;
sum = 15 + 5%10 = 15 + 5 = 20
sum = 20
Program
#include<stdio.h>
#include<conio.h>
void main()
{
long int num, sum = 0;
int d1, d2, d3, d4, d5;
printf("Enter a five digit number\t:");
scanf("%ld", &num);
d1 = num / 10000;
d2 = (num / 1000) % 10;
d3 = (num / 100) % 10;
d4 = (num / 10) % 10;
d5 = num % 10;
sum = d1 + d2 + d3 + d4 + d5;
printf("\nSum of digits = %d",sum);
getch();
}
Output
Enter a five digit number :45678
Sum of digits = 30
Explanation
A five digit number is taken as an input from the user in the variable named 'num'. %ld is the format specifier used for long int data type. A variable named 'sum' is used to carry the sum of the digits of the number. sum is initialized to 0.
The concept used is to extract all the digits of a number separately one by one and storing them in their respective variables. variables named 'd1', 'd2', 'd3', 'd4' and 'd5' are used for this purpose. The individual digits are all added and stored in the variable 'sum'.
To understand the concept, let us consider an example:
num = 45678
All the digits are extracted, now,
sum = d1 + d2 + d3 + d4 + d5
= 4 + 5 + 6 + 7 + 8
= 30
There is another way to extract the individual digits of the number. It is explained in the Method 3.
The concept used is to extract all the digits of a number separately one by one and storing them in their respective variables. variables named 'd1', 'd2', 'd3', 'd4' and 'd5' are used for this purpose. The individual digits are all added and stored in the variable 'sum'.
sum = d1 + d2 + d3 + d4 + d5
To understand the concept, let us consider an example:
num = 45678
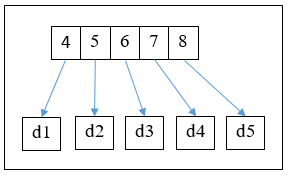
d1 = num / 10000;
d1 = 45678/10000 = 4
d2 = (num / 1000) % 10;
d2 = (45678/1000) % 10 = 45 % 10 = 5
d3 = (num / 100) % 10;
d3 = (45678/100) % 10 = 456 % 10 = 6
d4 = (num / 10) % 10;
d4 = (45678/10) % 10 = 4567 % 10 = 7
d5 = num % 10;
d5 = 45678 % 10 = 8
All the digits are extracted, now,
sum = d1 + d2 + d3 + d4 + d5
= 4 + 5 + 6 + 7 + 8
= 30
There is another way to extract the individual digits of the number. It is explained in the Method 3.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
long int num, sum = 0;
int d1, d2, d3, d4, d5;
printf("Enter a five digit number\t:");
scanf("%ld", &num);
d1 = num / 10000;
d2 = (num % 10000) / 1000;
d3 = (num % 1000) / 100;
d4 = (num % 100) / 10;
d5 = num % 10;
sum = d1 + d2 + d3 + d4 + d5;
printf("\nSum of digits = %d",sum);
getch();
}
Output
Enter a five digit number :23456
Sum of digits = 20
Explanation
In this program, the digits are extracted using the below logic.
To understand the concept, let us consider an example:
num = 23456
All the digits are extracted, now,
sum = d1 + d2 + d3 + d4 + d5
= 2 + 3 + 4 + 5 + 6
= 20
To understand the concept, let us consider an example:
num = 23456
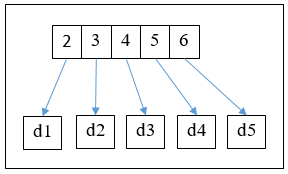
d1 = num / 10000;
d1 = 23456 / 10000 = 2
d2 = (num % 10000) / 1000;
d2 = (23456%10000) / 1000 = 3456 / 1000 = 3
d3 = (num % 1000) / 100;
d3 = (23456%1000) / 100 = 456 / 100 = 4
d4 = (num % 100) / 10;
d4 = (23456%100) / 10 = 56 / 10 = 5
d5 = num % 10;
d5 = 23456 % 10 = 6
All the digits are extracted, now,
sum = d1 + d2 + d3 + d4 + d5
= 2 + 3 + 4 + 5 + 6
= 20