Simple Interest
C program to calculate simple interest.
Program
#include<stdio.h>
#include<conio.h>
void main()
{
float p, r, t, si;
printf("Enter principal (Amount) : ");
scanf("%f",&p);
printf("Enter rate of interest per annum : ");
scanf("%f",&r);
printf("Enter time (in years) : ");
scanf("%f",&t);
si=(p*r*t)/100;
printf("\nSimple Interest = %f",si);
getch();
}
Output
Enter principal (Amount) : 2545
Enter rate of interest per annum : 3
Enter time (in years) : 2
Simple Interest = 152.699997
Explanation
In the above program, we are reading the values for principal amount, rate of interest per annum and time using the ‘p’, ‘r’ and ‘t’ variables respectively.
To find the simple interest, the following formula is used:
To find the simple interest, the following formula is used:
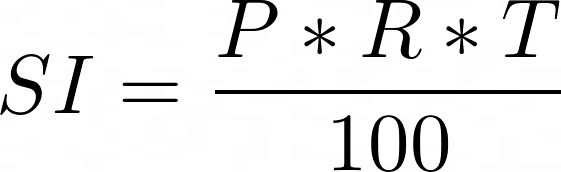