Find the saddle point of the matrix
C program to find the saddle point of the matrix.
saddle point is an element of the matrix, which is the minimum element in its row and the maximum in its column.
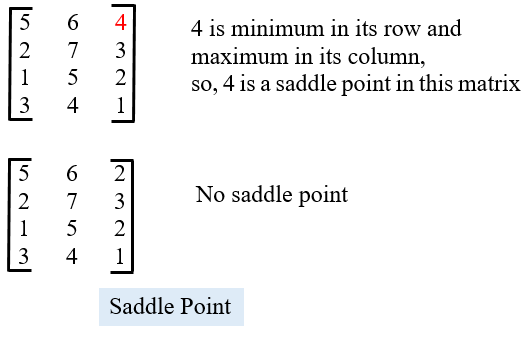
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void saddle(int mat[MAX][MAX], int row, int col)
{
int i, j, k;
int row_min, col_index, flag;
flag = 0;
for(i=0;i<row;i++)
{
row_min = mat[i][0];
col_index = 0;
for(j=1;j<col;j++)
{
if(row_min > mat[i][j])
{
row_min = mat[i][j];
col_index = j;
}
}
for(j=0;j<row;j++)
{
if(row_min < mat[j][col_index])
{
break;
}
}
if(j==row)
{
printf("\nSaddle point %d found at position (%d,%d)",row_min,i,col_index);
flag = 1;
}
}
if(flag == 0)
{
printf("\nThere is no saddle point");
}
}
void main()
{
int mat[MAX][MAX], mat1[MAX][MAX];
int row, col;
printf("Enter the number of rows\t:");
scanf("%d",&row);
printf("Enter the number of columns\t:");
scanf("%d",&col);
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
saddle(mat, row, col);
getch();
}
Output
********** Run1 **********
Enter the number of rows :3
Enter the number of columns :4
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [1][0] :1
Element [1][1] :5
Element [1][2] :6
Element [1][3] :7
Element [2][0] :1
Element [2][1] :8
Element [2][2] :9
Element [2][3] :0
Matrix entered is
1 2 3 4
1 5 6 7
1 8 9 0
Saddle point 1 found at position (0,0)
Saddle point 1 found at position (1,0)
********** Run2 **********
Enter the number of rows :3
Enter the number of columns :4
Enter the elements of the matrix
Element [0][0] :3
Element [0][1] :10
Element [0][2] :6
Element [0][3] :2
Element [1][0] :18
Element [1][1] :24
Element [1][2] :17
Element [1][3] :6
Element [2][0] :15
Element [2][1] :21
Element [2][2] :10
Element [2][3] :8
Matrix entered is
3 10 6 2
18 24 17 6
15 21 10 8
Saddle point 8 found at position (2,3)
********** Run3 **********
Enter the number of rows :3
Enter the number of columns :3
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :10
Element [2][1] :18
Element [2][2] :4
Matrix entered is
1 2 3
4 5 6
10 18 4
There is no saddle point