Print a matrix in z form
C program to print a matrix in z form.
Z form is only defined in square matrices.
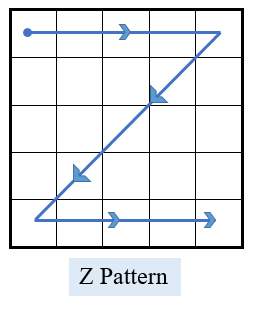
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void print_zpattern(int mat[MAX][MAX], int row)
{
int i;
for(i=0;i<row-1;i++)
{
printf("%d ",mat[0][i]);
}
for(i=row-1;i>=0;i--)
{
printf("%d ",mat[row-i-1][i]);
}
for(i=1;i<row;i++)
{
printf("%d ",mat[row-1][i]);
}
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
printf("\nMatrix in the Z form is\n");
print_zpattern(mat, row);
getch();
}
Output
Enter the number of rows or columns of the square matrix :5
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [0][4] :5
Element [1][0] :6
Element [1][1] :7
Element [1][2] :8
Element [1][3] :9
Element [1][4] :12
Element [2][0] :23
Element [2][1] :34
Element [2][2] :45
Element [2][3] :56
Element [2][4] :67
Element [3][0] :78
Element [3][1] :89
Element [3][2] :90
Element [3][3] :11
Element [3][4] :21
Element [4][0] :32
Element [4][1] :43
Element [4][2] :54
Element [4][3] :65
Element [4][4] :76
Matrix entered is
1 2 3 4 5
6 7 8 9 12
23 34 45 56 67
78 89 90 11 21
32 43 54 65 76
Matrix in the Z form is
1 2 3 4 5 9 45 89 32 43 54 65 76