Matrix is Binary matrix or not
C program to check if a matrix is Binary matrix or not.
A Binary Matrix is a matrix in which all the elements are either 0 or 1. No other element except 1 and 0 is allowed.
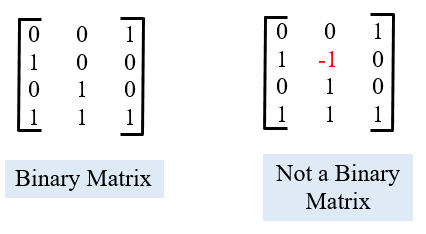
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
int binary(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
if(!(mat[i][j] == 0 || mat[i][j] == 1))
{
return(0);
}
}
}
return(1);
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows\t:");
scanf("%d",&row);
printf("Enter the number of columns\t:");
scanf("%d",&col);
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
if(binary(mat, row, col))
printf("\nMatrix is a binary matrix");
else
printf("\nMatrix is not a binary matrix");
getch();
}
Output
********** Run1 **********
Enter the number of rows :3
Enter the number of columns :4
Enter the elements of the matrix
Element [0][0] :0
Element [0][1] :1
Element [0][2] :1
Element [0][3] :0
Element [1][0] :0
Element [1][1] :1
Element [1][2] :1
Element [1][3] :1
Element [2][0] :1
Element [2][1] :0
Element [2][2] :0
Element [2][3] :0
Matrix entered is
0 1 1 0
0 1 1 1
1 0 0 0
Matrix is a binary matrix
********** Run2 **********
Enter the number of rows :3
Enter the number of columns :4
Enter the elements of the matrix
Element [0][0] :11
Element [0][1] :1
Element [0][2] :1
Element [0][3] :0
Element [1][0] :0
Element [1][1] :0
Element [1][2] :1
Element [1][3] :1
Element [2][0] :1
Element [2][1] :0
Element [2][2] :0
Element [2][3] :0
Matrix entered is
11 1 1 0
0 0 1 1
1 0 0 0
Matrix is not a binary matrix