Matrix is involutory matrix or not.
C program to check whether matrix is involutory matrix or not.
A matrix is said to be an involutory matrix if the multiplication of that matrix with itself results an identity matrix. i.e. a matrix A is said to be an Involutory matrix if and only if A*A = I. where I is an identity matrix.
Note: it is important that an involutory matrix should be a square matrix. Because for the multiplication of two matrices the number of rows of the first matrix should be equal to the number of columns of the second matrix. so, for multiplication of a matrix with itself, it is only possible when a matrix is a square matrix.
Note: it is important that an involutory matrix should be a square matrix. Because for the multiplication of two matrices the number of rows of the first matrix should be equal to the number of columns of the second matrix. so, for multiplication of a matrix with itself, it is only possible when a matrix is a square matrix.
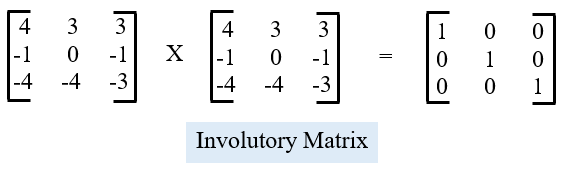
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void multiply(int mat[MAX][MAX], int mat1[MAX][MAX], int mul[MAX][MAX], int row)
{
int i, j, k;
for(i=0;i<row;i++)
{
for(j=0;j<row;j++)
{
mul[i][j] = 0;
for(k=0;k<row;k++)
{
mul[i][j] = mul[i][j] + (mat[i][k] * mat1[k][j]);
}
}
}
}
int identity(int mat[MAX][MAX], int row)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<row;j++)
{
if((i==j) && mat[i][j]!=1.0)
{
return(0);
}
else if(i!=j && mat[i][j]!=0.0)
{
return(0);
}
}
}
return(1);
}
int involutory(int mat[MAX][MAX], int row)
{
int i, j, flag, mul[MAX][MAX];
multiply(mat, mat, mul, row);
flag = identity(mul, row);
return(flag);
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
if(involutory(mat, row))
printf("\nMatrix is an involutory matrix");
else
printf("\nMatrix is not an involutory matrix");
getch();
}
Output
********** Run1 **********
Enter the number of rows or columns of the square matrix :3
Enter the elements of the matrix
Element [0][0] :-5
Element [0][1] :-8
Element [0][2] :0
Element [1][0] :3
Element [1][1] :5
Element [1][2] :0
Element [2][0] :1
Element [2][1] :2
Element [2][2] :-1
Matrix entered is
-5 -8 0
3 5 0
1 2 -1
Matrix is an involutory matrix
********** Run2 **********
Enter the number of rows or columns of the square matrix :3
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :7
Element [2][1] :8
Element [2][2] :9
Matrix entered is
1 2 3
4 5 6
7 8 9
Matrix is not an involutory matrix