Matrix is orthogonal or not
C program to check whether matrix is orthogonal or not.
An orthogonal matrix is a square matrix that satisfies the following condition:
A * AT = I (Identity Matrix)
So, A Matrix is an orthogonal matrix, when we multiply it with its transpose, we get an identity matrix.
A * AT = I (Identity Matrix)
So, A Matrix is an orthogonal matrix, when we multiply it with its transpose, we get an identity matrix.
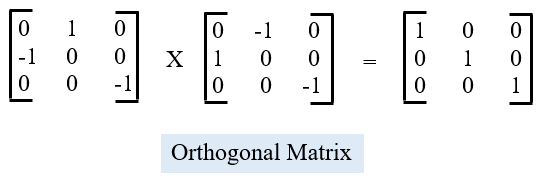
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void transpose(int mat[MAX][MAX], int trans[MAX][MAX], int row)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<row;j++)
{
trans[j][i] = mat[i][j];
}
}
}
void multiply(int mat[MAX][MAX], int trans[MAX][MAX], int mul[MAX][MAX], int row)
{
int i, j, k;
for(i=0;i<row;i++)
{
for(j=0;j<row;j++)
{
mul[i][j] = 0;
for(k=0;k<row;k++)
{
mul[i][j] = mul[i][j] + (mat[i][k] * trans[k][j]);
}
}
}
}
void identity(int mat[MAX][MAX], int row)
{
int i, j, flag;
flag = 0;
for(i=0;i<row;i++)
{
for(j=0;j<row;j++)
{
if((i==j) && mat[i][j]!=1.0)
{
flag = 1;
}
else if(i!=j && mat[i][j]!=0.0)
{
flag = 1;
}
}
}
if(flag == 0)
{
printf("\nMatrix is orthogonal matrix");
}
else
{
printf("\nMatrix is not an orthogonal matrix");
}
}
void orthogonal(int mat[MAX][MAX], int row)
{
int i, j;
int trans[MAX][MAX], mul[MAX][MAX];
transpose(mat, trans, row);
multiply(mat, trans, mul, row);
identity(mul, row);
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
orthogonal(mat, row);
getch();
}
Output
********** Run1 **********
Enter the number of rows or columns of the square matrix :4
Enter the elements of the matrix
Element [0][0] :0
Element [0][1] :0
Element [0][2] :0
Element [0][3] :1
Element [1][0] :0
Element [1][1] :0
Element [1][2] :1
Element [1][3] :0
Element [2][0] :1
Element [2][1] :0
Element [2][2] :0
Element [2][3] :0
Element [3][0] :0
Element [3][1] :1
Element [3][2] :0
Element [3][3] :0
Matrix entered is
0 0 0 1
0 0 1 0
1 0 0 0
0 1 0 0
Matrix is orthogonal matrix
********** Run2 **********
Enter the number of rows or columns of the square matrix :4
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :0
Element [0][2] :0
Element [0][3] :0
Element [1][0] :0
Element [1][1] :0
Element [1][2] :1
Element [1][3] :0
Element [2][0] :0
Element [2][1] :1
Element [2][2] :0
Element [2][3] :0
Element [3][0] :0
Element [3][1] :1
Element [3][2] :0
Element [3][3] :0
Matrix entered is
1 0 0 0
0 0 1 0
0 1 0 0
0 1 0 0
Matrix is not an orthogonal matrix