Matrix is a magic square or not
C program to check whether matrix is magic square or not.
A Magic Square is a n x n square matrix of distinct element from 1 to n2 where sum of any row, column or diagonal is always equal to same number.
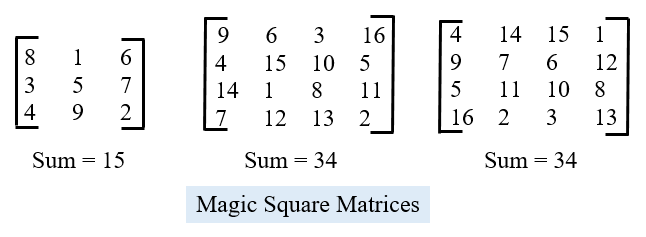
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
int magic_square(int mat[MAX][MAX], int row)
{
int i, j, diag_sum, diag_sum1, row_sum, col_sum;
diag_sum = 0;
for(i=0;i<row;i++)
{
diag_sum = diag_sum + mat[i][i];
}
diag_sum1 = 0;
for(i=0;i<row;i++)
{
diag_sum1 = diag_sum1 + mat[i][row-i-1];
}
if(diag_sum != diag_sum1)
return (0);
for(i=0;i<row;i++)
{
row_sum = 0;
for(j=0;j<row;j++)
{
row_sum = row_sum + mat[i][j];
}
if(row_sum != diag_sum)
return(0);
}
for(i=0;i<row;i++)
{
col_sum = 0;
for(j=0;j<row;j++)
{
col_sum = col_sum + mat[j][i];
}
if(col_sum != diag_sum)
return(0);
}
return(1);
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
if(magic_square(mat, row))
printf("\nMatrix is magic square");
else
printf("\nMatrix is not a magic square");
getch();
}
Output
********** Run1 **********
Enter the number of rows or columns of the square matrix :3
Enter the elements of the matrix
Element [0][0] :2
Element [0][1] :7
Element [0][2] :6
Element [1][0] :9
Element [1][1] :5
Element [1][2] :1
Element [2][0] :4
Element [2][1] :3
Element [2][2] :8
Matrix entered is
2 7 6
9 5 1
4 3 8
Matrix is magic square
********** Run2 **********
Enter the number of rows or columns of the square matrix :3
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :2
Element [1][0] :2
Element [1][1] :2
Element [1][2] :1
Element [2][0] :2
Element [2][1] :1
Element [2][2] :2
Matrix entered is
1 2 2
2 2 1
2 1 2
Matrix is not a magic square