Matrix is lower triangular or not
C program to check if matrix is lower triangular or not.
A square matrix is called lower triangular if all the entries above the main diagonal are zero.
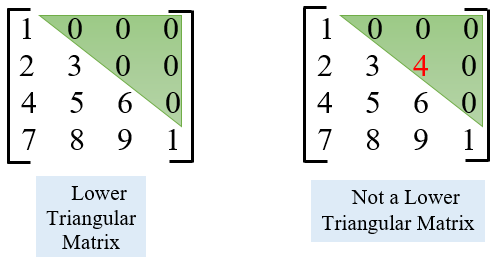
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void low_tri(int mat[MAX][MAX], int row)
{
int i, j, flag;
flag = 0;
for(i=0;i<row;i++)
{
for(j=i+1;j<row;j++)
{
if(mat[i][j] != 0)
{
flag = 1;
}
}
}
if(flag == 0)
{
printf("\nMatrix is lower triangular");
}
else
{
printf("\nMatrix is not lower triangular");
}
}
void main()
{
int mat[MAX][MAX];
int row, col;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements of the matrix\n");
read_matrix(mat, row, col);
printf("\nMatrix entered is\n");
print_matrix(mat, row, col);
low_tri(mat, row);
getch();
}
Output
********** Run1 **********
Enter the number of rows or columns of the square matrix :4
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :0
Element [0][2] :0
Element [0][3] :0
Element [1][0] :2
Element [1][1] :3
Element [1][2] :0
Element [1][3] :0
Element [2][0] :4
Element [2][1] :5
Element [2][2] :6
Element [2][3] :0
Element [3][0] :7
Element [3][1] :8
Element [3][2] :9
Element [3][3] :10
Matrix entered is
1 0 0 0
2 3 0 0
4 5 6 0
7 8 9 10
Matrix is lower triangular
********** Run2 **********
Enter the number of rows or columns of the square matrix :4
Enter the elements of the matrix
Element [0][0] :1
Element [0][1] :0
Element [0][2] :2
Element [0][3] :0
Element [1][0] :3
Element [1][1] :4
Element [1][2] :0
Element [1][3] :0
Element [2][0] :5
Element [2][1] :6
Element [2][2] :7
Element [2][3] :0
Element [3][0] :8
Element [3][1] :9
Element [3][2] :10
Element [3][3] :11
Matrix entered is
1 0 2 0
3 4 0 0
5 6 7 0
8 9 10 11
Matrix is not lower triangular