Two matrices equal or not
C program to check whether two matrices are equal or not.
Two matrices are said to be equal if and only if they satisfy the following conditions:
Both the matrices should have the same number of rows and columns.
Both the matrices should have the same corresponding elements.
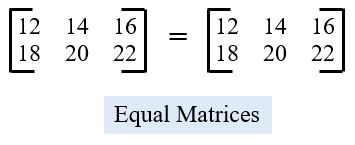
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void equal(int mat1[MAX][MAX], int mat2[MAX][MAX], int row1, int col1)
{
int i, j, flag;
flag = 0;
for(i=0;i<row1;i++)
{
for(j=0;j<col1;j++)
{
if(mat1[i][j] != mat2[i][j])
{
flag = 1;
break;
}
}
}
if(flag == 0)
{
printf("\nMatrices are equal");
}
else
{
printf("\nMatrices are not equal");
}
}
void main()
{
int mat1[MAX][MAX], mat2[MAX][MAX];
int row1, col1, row2, col2;
printf("Enter the number of rows of first matrix\t:");
scanf("%d",&row1);
printf("Enter the number of columns of first matrix\t:");
scanf("%d",&col1);
printf("Enter the number of rows of second matrix\t:");
scanf("%d",&row2);
printf("Enter the number of columns of second matrix\t:");
scanf("%d",&col2);
if(row1==row2 && col1==col2)
{
printf("Enter the elements of the first matrix\n");
read_matrix(mat1, row1, col1);
printf("Enter the elements of the second matrix\n");
read_matrix(mat2, row2, col2);
printf("\nFirst matrix entered is\n");
print_matrix(mat1, row1, col1);
printf("\nSecond matrix entered is\n");
print_matrix(mat2, row2, col2);
equal(mat1, mat2, row1, col1);
}
else
{
printf("\nMatrices cannot be compared");
}
getch();
}
Output
********** Run1 **********
Enter the number of rows of first matrix :2
Enter the number of columns of first matrix :3
Enter the number of rows of second matrix :3
Enter the number of columns of second matrix :4
Matrices cannot be compared
********** Run2 **********
Enter the number of rows of first matrix :2
Enter the number of columns of first matrix :3
Enter the number of rows of second matrix :2
Enter the number of columns of second matrix :3
Enter the elements of the first matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Enter the elements of the second matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
First matrix entered is
1 2 3
4 5 6
Second matrix entered is
1 2 3
4 5 6
Matrices are equal
********** Run3 **********
Enter the number of rows of first matrix :2
Enter the number of columns of first matrix :3
Enter the number of rows of second matrix :2
Enter the number of columns of second matrix :3
Enter the elements of the first matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Enter the elements of the second matrix
Element [0][0] :1
Element [0][1] :1
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
First matrix entered is
1 2 3
4 5 6
Second matrix entered is
1 1 3
4 5 6
Matrices are not equal