Display upper and lower triangle of the matrix
C program to display upper and lower triangle of the matrix.
Lower Triangular Matrix
A square matrix is called lower triangular if all the entries above the main diagonal are zero.
Upper Triangular Matrix
A square matrix is called upper triangular if all the entries below the main diagonal are zero.
A square matrix is called lower triangular if all the entries above the main diagonal are zero.
Upper Triangular Matrix
A square matrix is called upper triangular if all the entries below the main diagonal are zero.
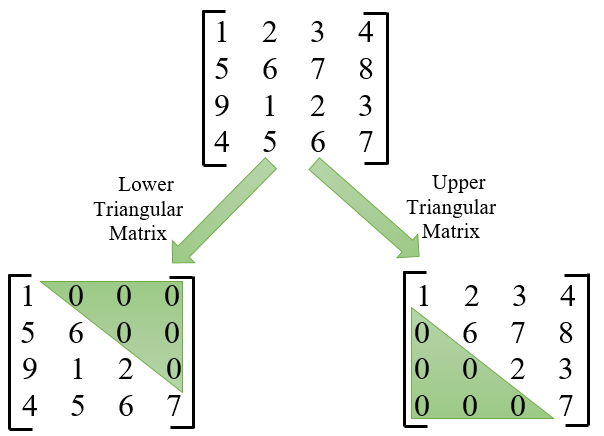
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void main()
{
int mat[MAX][MAX];
int row, col;
int i, j;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
printf("\n\nMatrix entered is\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
printf("\nLower Triangular Matrix\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
if(i<j)
{
printf("0\t");
}
else
{
printf("%d\t",mat[i][j]);
}
}
printf("\n");
}
printf("\nUpper Triangular Matrix\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
if(i>j)
{
printf("0\t");
}
else
{
printf("%d\t",mat[i][j]);
}
}
printf("\n");
}
getch();
}
Output
Enter the number of rows or columns of the square matrix :4
Enter the elements
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [1][0] :5
Element [1][1] :6
Element [1][2] :7
Element [1][3] :8
Element [2][0] :9
Element [2][1] :10
Element [2][2] :11
Element [2][3] :12
Element [3][0] :13
Element [3][1] :14
Element [3][2] :15
Element [3][3] :16
Matrix entered is
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
Lower Triangular Matrix
1 0 0 0
5 6 0 0
9 10 11 0
13 14 15 16
Upper Triangular Matrix
1 2 3 4
0 6 7 8
0 0 11 12
0 0 0 16