Find trace of a matrix
C program to find trace of a matrix.
Trace is defined only for square matrix (number of rows = number of columns). Trace is calculated by adding up the main diagonal elements of the matrix. The main diagonal elements are the ones that occur from top left of matrix down to bottom right corner. Elements in main diagonal have same indices for row and column.
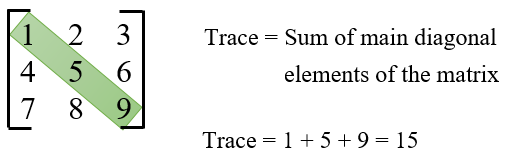
Program
#include<stdio.h>
#include<conio.h>
#define MAX
void main()
{
int mat[MAX][MAX];
int row, col, trace;
int i, j;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
printf("\n\nMatrix entered is\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
trace = 0;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
if(i==j)
{
trace = trace + mat[i][j];
}
}
}
printf("\nTrace of the matrix is %d",trace);
getch();
}
Output
Enter the number of rows or columns of the square matrix :4
Enter the elements
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [1][0] :5
Element [1][1] :6
Element [1][2] :7
Element [1][3] :8
Element [2][0] :9
Element [2][1] :10
Element [2][2] :11
Element [2][3] :12
Element [3][0] :13
Element [3][1] :14
Element [3][2] :15
Element [3][3] :16
Matrix entered is
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
Trace of the matrix is 34