Find normal of a square matrix.
C program to find normal of a square matrix.
Normal is defined only for square matrix (number of rows = number of columns). Normal of the matrix is the square root of the sum of the squares of each element of the matrix.
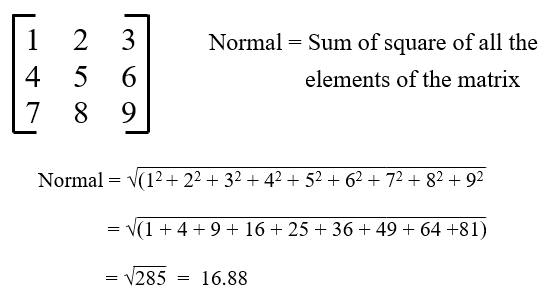
Program
#include<stdio.h>
#include<conio.h>
#include<math.h>
#define MAX 10
void main()
{
int mat[MAX][MAX];
int row, col, sum;
double normal;
int i, j;
printf("Enter the number of rows or columns of the square matrix\t:");
scanf("%d",&row);
col = row;
printf("Enter the elements\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
printf("\n\nMatrix entered is\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
sum = 0;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
sum = sum + (mat[i][j]*mat[i][j]);
}
}
normal = sqrt(sum);
printf("\nNormal of the matrix is %lf",normal);
getch();
}
Output
Enter the number of rows or columns of the square matrix :3
Enter the elements
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :7
Element [2][1] :8
Element [2][2] :9
Matrix entered is
1 2 3
4 5 6
7 8 9
Normal of the matrix is 16.881943