Multiply 'N' matrices
C program to multiply āNā matrices.
To multiply two matrices, the number of columns of the first matrix should be equal to the number of rows of the second matrix.
As a result of multiplication, a new matrix is obtained that has the same quantity of rows as the 1st one has and the same quantity of columns as the 2nd one.
For example, if you multiply a matrix of 'n' x 'k' by 'k' x 'm' size you'll get a new one of 'n' x 'm' dimension.
For 'N' matrices, matrices are multiplied one by one and number of columns of the resultant matrix must be equal to the number of rows of the next matrix to be multiplied.
To multiply two matrices, multiply the elements of each row of the first matrix by the elements of each column in the second matrix and add the products.
For example,
As a result of multiplication, a new matrix is obtained that has the same quantity of rows as the 1st one has and the same quantity of columns as the 2nd one.
For example, if you multiply a matrix of 'n' x 'k' by 'k' x 'm' size you'll get a new one of 'n' x 'm' dimension.
For 'N' matrices, matrices are multiplied one by one and number of columns of the resultant matrix must be equal to the number of rows of the next matrix to be multiplied.
To multiply two matrices, multiply the elements of each row of the first matrix by the elements of each column in the second matrix and add the products.
For example,
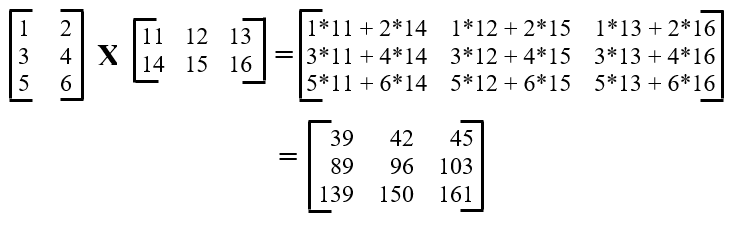
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void mul_matrix(int mat1[MAX][MAX], int mat2[MAX][MAX], int mul[MAX][MAX], int row1, int col1, int col2)
{
int i, j, k;
for(i=0;i<row1;i++)
{
for(j=0;j<col2;j++)
{
mul[i][j] = 0;
for(k=0;k<col1;k++)
{
mul[i][j] = mul[i][j] + (mat1[i][k] * mat2[k][j]);
}
}
}
}
void main()
{
int mat1[MAX][MAX], mat2[MAX][MAX], mul[MAX][MAX];
int row1, col1, row2, col2, flag, i, j;
char ch;
printf("Enter the number of rows of first matrix\t:");
scanf("%d",&row1);
printf("Enter the number of columns of second matrix\t:");
scanf("%d",&col1);
printf("Enter the elements of first matrix\n");
read_matrix(mat1, row1, col1);
flag = 0;
do
{
if(flag == 1)
{
for(i=0;i<row1;i++)
{
for(j=0;j<col2;j++)
{
mat1[i][j] = mul[i][j];
}
}
row1 = row1;
col1 = col2;
}
printf("\nEnter the number of rows of another matrix\t:");
scanf("%d",&row2);
printf("Enter the number of columns of another matrix\t:");
scanf("%d",&col2);
if(col1 == row2)
{
printf("\nEnter the elements of another matrix\n");
read_matrix(mat2, row2, col2);
printf("\nFirst matrix is\n");
print_matrix(mat1, row1, col1);
printf("\nSecond matrix is\n");
print_matrix(mat2, row2, col2);
mul_matrix(mat1, mat2, mul, row1, col1, col2);
printf("\nMatrix after multiplication\n");
print_matrix(mul, row1, col2);
printf("\nDo you want to multiply another matrix in the result (y/n)\t:");
fflush(stdin);
scanf("%c",&ch);
flag = 1;
}
else
{
printf("\nMatrices of this order cannot be multiplied");
printf("\nDo you want to multiply another matrix in the result (y/n)\t:");
fflush(stdin);
scanf("%c",&ch);
flag = 1;
}
}
while(ch == 'y' || ch == 'Y');
getch();
}
Output
Enter the number of rows of first matrix :3
Enter the number of columns of second matrix :3
Enter the elements of first matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :7
Element [2][1] :8
Element [2][2] :9
Enter the number of rows of another matrix :3
Enter the number of columns of another matrix :4
Enter the elements of another matrix
Element [0][0] :5
Element [0][1] :4
Element [0][2] :3
Element [0][3] :2
Element [1][0] :1
Element [1][1] :3
Element [1][2] :4
Element [1][3] :5
Element [2][0] :6
Element [2][1] :7
Element [2][2] :8
Element [2][3] :9
First matrix is
1 2 3
4 5 6
7 8 9
Second matrix is
5 4 3 2
1 3 4 5
6 7 8 9
Matrix after multiplication
25 31 35 39
61 73 80 87
97 115 125 135
Do you want to multiply another matrix in the result (y/n) :y
Enter the number of rows of another matrix :3
Enter the number of columns of another matrix :4
Matrices of this order cannot be multiplied
Do you want to multiply another matrix in the result (y/n) :y
Enter the number of rows of another matrix :4
Enter the number of columns of another matrix :2
Enter the elements of another matrix
Element [0][0] :1
Element [0][1] :2
Element [1][0] :3
Element [1][1] :4
Element [2][0] :4
Element [2][1] :3
Element [3][0] :2
Element [3][1] :1
First matrix is
25 31 35 39
61 73 80 87
97 115 125 135
Second matrix is
1 2
3 4
4 3
2 1
Matrix after multiplication
336 318
774 741
1212 1164
Do you want to multiply another matrix in the result (y/n) :n