Add 'N' matrices
C program to add āNā matrices.
'N' matrices may be added if and only if they all have the same dimension; i.e. they must have the same number of rows and columns.
Addition is accomplished by adding corresponding elements. For example,
Addition is accomplished by adding corresponding elements. For example,
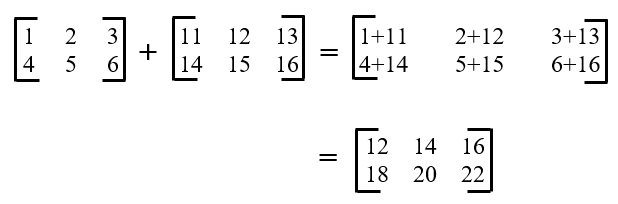
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void add_matrix(int mat1[MAX][MAX], int mat2[MAX][MAX], int add[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
add[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
void main()
{
int mat1[MAX][MAX], mat2[MAX][MAX], add[MAX][MAX];
int row, col, flag, i, j;
char ch;
printf("Enter the number of rows\t:");
scanf("%d",&row);
printf("Enter the number of columns\t:");
scanf("%d",&col);
printf("Enter the elements of first matrix\n");
read_matrix(mat1, row, col);
flag = 0;
do
{
printf("\nEnter the elements of another matrix\n");
read_matrix(mat2, row, col);
if(flag == 1)
{
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
mat1[i][j] = add[i][j];
}
}
}
printf("\nFirst matrix is\n");
print_matrix(mat1, row, col);
printf("\nSecond matrix is\n");
print_matrix(mat2, row, col);
add_matrix(mat1, mat2, add, row, col);
printf("\nMatrix after addition\n");
print_matrix(add, row, col);
printf("\nDo you want to add another matrix in the result (y/n)\t:");
fflush(stdin);
scanf("%c",&ch);
flag = 1;
}
while(ch == 'y' || ch == 'Y');
getch();
}
Output
Enter the number of rows :3
Enter the number of columns :3
Enter the elements of first matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :7
Element [2][1] :8
Element [2][2] :9
Enter the elements of another matrix
Element [0][0] :7
Element [0][1] :6
Element [0][2] :5
Element [1][0] :8
Element [1][1] :9
Element [1][2] :4
Element [2][0] :3
Element [2][1] :2
Element [2][2] :1
First matrix is
1 2 3
4 5 6
7 8 9
Second matrix is
7 6 5
8 9 4
3 2 1
Matrix after addition
8 8 8
12 14 10
10 10 10
Do you want to add another matrix in the result (y/n) :y
Enter the elements of another matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [1][0] :4
Element [1][1] :5
Element [1][2] :6
Element [2][0] :7
Element [2][1] :8
Element [2][2] :9
First matrix is
8 8 8
12 14 10
10 10 10
Second matrix is
1 2 3
4 5 6
7 8 9
Matrix after addition
9 10 11
16 19 16
17 18 19
Do you want to add another matrix in the result (y/n) :y
Enter the elements of another matrix
Element [0][0] :3
Element [0][1] :4
Element [0][2] :5
Element [1][0] :6
Element [1][1] :7
Element [1][2] :8
Element [2][0] :9
Element [2][1] :2
Element [2][2] :1
First matrix is
9 10 11
16 19 16
17 18 19
Second matrix is
3 4 5
6 7 8
9 2 1
Matrix after addition
12 14 16
22 26 24
26 20 20
Do you want to add another matrix in the result (y/n) :n