Addition and subtraction of two matrices
C program to find Addition and subtraction of two matrices.
Two matrices may be added or subtracted only if they have the same dimension; i.e. they must have the same number of rows and columns.
Addition or subtraction is accomplished by adding or subtracting corresponding elements. For example,
Addition or subtraction is accomplished by adding or subtracting corresponding elements. For example,
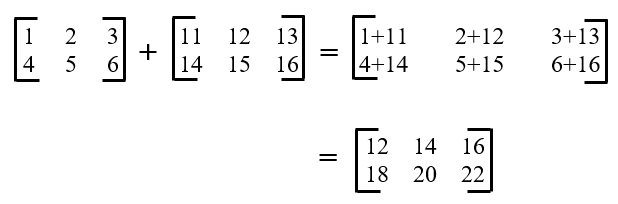
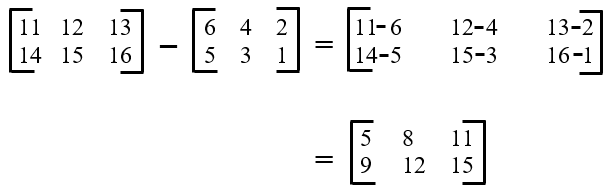
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void read_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
}
void print_matrix(int mat[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
}
void add_matrix(int mat1[MAX][MAX], int mat2[MAX][MAX], int add[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
add[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
void sub_matrix(int mat1[MAX][MAX], int mat2[MAX][MAX], int sub[MAX][MAX], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
sub[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
void main()
{
int mat1[MAX][MAX], mat2[MAX][MAX], add[MAX][MAX], sub[MAX][MAX];
int row, col;
printf("Enter the number of rows\t:");
scanf("%d",&row);
printf("Enter the number of columns\t:");
scanf("%d",&col);
printf("Enter the elements of first matrix\n");
read_matrix(mat1, row, col);
printf("Enter the elements of second matrix\n");
read_matrix(mat2, row, col);
printf("\nFirst matrix entered is\n");
print_matrix(mat1, row, col);
printf("\nSecond matrix entered is\n");
print_matrix(mat2, row, col);
add_matrix(mat1, mat2, add, row, col);
sub_matrix(mat1, mat2, sub, row, col);
printf("\nMatrix after addition\n");
print_matrix(add, row, col);
printf("\nMatrix after subtraction\n");
print_matrix(sub, row, col);
getch();
}
Output
Enter the number of rows :3
Enter the number of columns :4
Enter the elements of first matrix
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [1][0] :4
Element [1][1] :3
Element [1][2] :2
Element [1][3] :1
Element [2][0] :5
Element [2][1] :6
Element [2][2] :7
Element [2][3] :8
Enter the elements of second matrix
Element [0][0] :8
Element [0][1] :7
Element [0][2] :6
Element [0][3] :5
Element [1][0] :9
Element [1][1] :8
Element [1][2] :6
Element [1][3] :5
Element [2][0] :4
Element [2][1] :3
Element [2][2] :5
Element [2][3] :2
First matrix entered is
1 2 3 4
4 3 2 1
5 6 7 8
Second matrix entered is
8 7 6 5
9 8 6 5
4 3 5 2
Matrix after addition
9 9 9 9
13 11 8 6
9 9 12 10
Matrix after subtraction
-7 -5 -3 -1
-5 -5 -4 -4
1 3 2 6