Transpose of matrix
C program to transpose a matrix.
The transpose of a given matrix is a new matrix formed by interchanging the rows and columns of a matrix. i.e. rows of the original matrix will become columns of the new matrix. Similarly, columns in the original matrix will become rows in the new matrix.
In other words, transpose of A[][] is obtained by changing A[i][j] to A[j][i].
When we transpose a matrix, its order changes (if original matrix is of the order 3x2, then new matrix will be of the order 2x3), but for a square matrix, it remains the same.
In other words, transpose of A[][] is obtained by changing A[i][j] to A[j][i].
When we transpose a matrix, its order changes (if original matrix is of the order 3x2, then new matrix will be of the order 2x3), but for a square matrix, it remains the same.
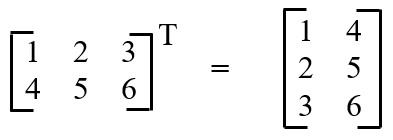
Program
#include<stdio.h>
#include<conio.h>
#define MAX 10
void main()
{
int mat[MAX][MAX], trans[MAX][MAX];
int row, col;
int i, j;
printf("Enter the number of rows\t:");
scanf("%d",&row);
printf("Enter the number of columns\t:");
scanf("%d",&col);
printf("Enter the elements\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("Element [%d][%d]\t:",i,j);
scanf("%d",&mat[i][j]);
}
}
printf("\n\nMatrix entered is\n");
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
printf("%d\t",mat[i][j]);
}
printf("\n");
}
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
{
trans[j][i] = mat[i][j];
}
}
printf("\n\nTranspose of the matrix is\n");
for(i=0;i<col;i++)
{
for(j=0;j<row;j++)
{
printf("%d\t",trans[i][j]);
}
printf("\n");
}
getch();
}
Output
Enter the number of rows :3
Enter the number of columns :4
Enter the elements
Element [0][0] :1
Element [0][1] :2
Element [0][2] :3
Element [0][3] :4
Element [1][0] :5
Element [1][1] :6
Element [1][2] :7
Element [1][3] :8
Element [2][0] :9
Element [2][1] :10
Element [2][2] :11
Element [2][3] :12
Matrix entered is
1 2 3 4
5 6 7 8
9 10 11 12
Transpose of the matrix is
1 5 9
2 6 10
3 7 11
4 8 12